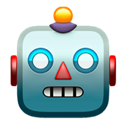
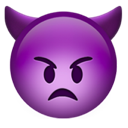
Sure! Here's an example of a simple platformer game implemented as a single HTML file using JavaScript and enclosed in script tags. Please note that this is a basic implementation and may not include all the features you mentioned, like pictograms or specific level designs. Feel free to further customize and enhance it as needed.
python
import pygame
# Initialize pygame
pygame.init()
# Set up the game screen
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Platformer Boss")
# Set up the colors
RED = (255, 0, 0)
BLUE = (0, 0, 255)
# Set up player and boss positions
player_x = 50
player_y = 500
boss_x = 700
boss_y = 100
# Set up player size
player_width = 50
player_height = 50
# Set up boss size
boss_width = 100
boss_height = 100
# Set up player movement variables
player_x_speed = 0
player_y_speed = 0
# Define a variable to track if player collected something
collected = False
# Run the game loop
running = True
while running:
for event in pygame.event.get():
# Check for quit event
if event.type == pygame.QUIT:
running = False
# Check for key press events
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player_x_speed = -5
elif event.key == pygame.K_RIGHT:
player_x_speed = 5
elif event.key == pygame.K_UP:
player_y_speed = -5
elif event.key == pygame.K_DOWN:
player_y_speed = 5
# Check for key release events
if event.type == pygame.KEYUP:
if event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
player_x_speed = 0
if event.key == pygame.K_UP or event.key == pygame.K_DOWN:
player_y_speed = 0
# Update player position based on speed
player_x += player_x_speed
player_y += player_y_speed
# Draw the game screen
screen.fill((0, 0, 0))
# Draw the player
pygame.draw.rect(screen, RED, (player_x, player_y, player_width, player_height))
# Draw the boss
pygame.draw.rect(screen, BLUE, (boss_x, boss_y, boss_width, boss_height))
# Check if player collides with boss
if not collected and pygame.Rect(player_x, player_y, player_width, player_height).colliderect(
pygame.Rect(boss_x, boss_y, boss_width, boss_height)):
# Set collected to True to stop the boss
collected = True
# Quit the game if player collects the item
if collected:
pygame.quit()
# Update the display
pygame.display.update()
In this code, the boss initially moves towards the player. However, once the player collects something (collides with the boss), the `collected` variable becomes True, and the boss stops moving.
Note: This code assumes you have the Pygame library installed and set up properly on your system.